class
simulationA soft-tissue simulation.
Constructors, destructors, conversion operators
- operator bool() const explicit noexcept
- Returns true if this simulation object is not 'null' (i.e. not default-constructed or moved-from).
- simulation() defaulted noexcept
- Default-constructs a 'null' simulation.
- simulation(simulation&& other) noexcept
- Move constructor.
- ~simulation() noexcept
- Destructor.
Public functions
- auto operator=(simulation&& rhs) -> simulation& noexcept
- Move-assignment operator.
- auto start() -> result noexcept
- Starts the simulation.
- auto update(duration timestep, duration max_solve_time = {}) -> result noexcept
- Advances the simulation.
Adding objects
-
auto add_object(const rigid_
body_ description& desc) -> result<rigid_ body_ handle> noexcept - Adds a new rigid body to the simulation.
-
auto add_object(const soft_
body_ description& desc) -> result<soft_ body_ handle> noexcept - Adds a new soft body to the simulation.
-
auto add_object(const volumetric_
body_ description& desc) -> result<volumetric_ body_ handle> noexcept - Adds a new volumetric body to the simulation.
-
auto add_object(const force_
feedback_ emitter_ description& desc) -> result<force_ feedback_ emitter_ handle> noexcept - Adds a new force-feedback emitter to the simulation.
Creating a simulation
-
static auto create(const simulation_
description& desc) -> result<simulation> noexcept - Creates a simulation.
Debugging and diagnostics
-
auto debug_draw(debug_
draw_ interface& dd, debug_ draw_ groups groups = debug_draw_groups:: defaults, size_t batch_index = 0, size_t batch_count = 1) const -> result noexcept - Draws debug geometry.
-
auto debug_dump_profiler() const -> result<string_
view> noexcept - Dumps out the last snapshot of the simulation's profiler timing data.
-
auto debug_ui(debug_
ui_ interface& ui, debug_ ui_ groups groups = debug_ui_groups:: defaults) -> result noexcept - Displays a debug user interface.
Function documentation
stim:: simulation:: simulation() defaulted noexcept
Default-constructs a 'null' simulation.
result stim:: simulation:: update(duration timestep,
duration max_solve_time = {}) noexcept
Advances the simulation.
Parameters | |
---|---|
timestep | The timestep to advance the simulation by. Cannot be negative. |
max_solve_time | The max real-world clock time that may elapse while trying to complete the physics solve. Cannot be negative. Leave as default to mean "unlimited time allowed". Setting a real value for this will cause update() to abort with an error if the internal solve takes more than this amount. |
Returns | A success result<> , or an error message detailing why the update failed. |
result<rigid_ body_ handle> stim:: simulation:: add_object(const rigid_ body_ description& desc) noexcept
Adds a new rigid body to the simulation.
Parameters | |
---|---|
desc | A description of the rigid body being added. |
Returns | A handle to the newly-created object, or an error message detailing why object creation failed. |
result<soft_ body_ handle> stim:: simulation:: add_object(const soft_ body_ description& desc) noexcept
Adds a new soft body to the simulation.
Parameters | |
---|---|
desc | A description of the soft body being added. |
Returns | A handle to the newly-created object, or an error message detailing why object creation failed. |
result<volumetric_ body_ handle> stim:: simulation:: add_object(const volumetric_ body_ description& desc) noexcept
Adds a new volumetric body to the simulation.
Parameters | |
---|---|
desc | A description of the volumetric body being added. |
Returns | A handle to the newly-created object, or an error message detailing why object creation failed. |
result<force_ feedback_ emitter_ handle> stim:: simulation:: add_object(const force_ feedback_ emitter_ description& desc) noexcept
Adds a new force-feedback emitter to the simulation.
Parameters | |
---|---|
desc | A description of the feedback emitter being added. |
Returns | A handle to the newly-created object, or an error message detailing why object creation failed. |
static result<simulation> stim:: simulation:: create(const simulation_ description& desc) noexcept
Creates a simulation.
Parameters | |
---|---|
desc | The simulation description. |
Returns | A new simulation, or an error message detailing why instantiation failed. |
result stim:: simulation:: debug_draw(debug_ draw_ interface& dd,
debug_ draw_ groups groups = debug_draw_groups:: defaults,
size_t batch_index = 0,
size_t batch_count = 1) const noexcept
Draws debug geometry.
Parameters | |
---|---|
dd | The debug_ |
groups | The groups to draw. |
batch_index | The batch index of this draw call. |
batch_count | The total number of draw batches. |
Returns | A success result<> , or an error message detailing why the draw call failed. |
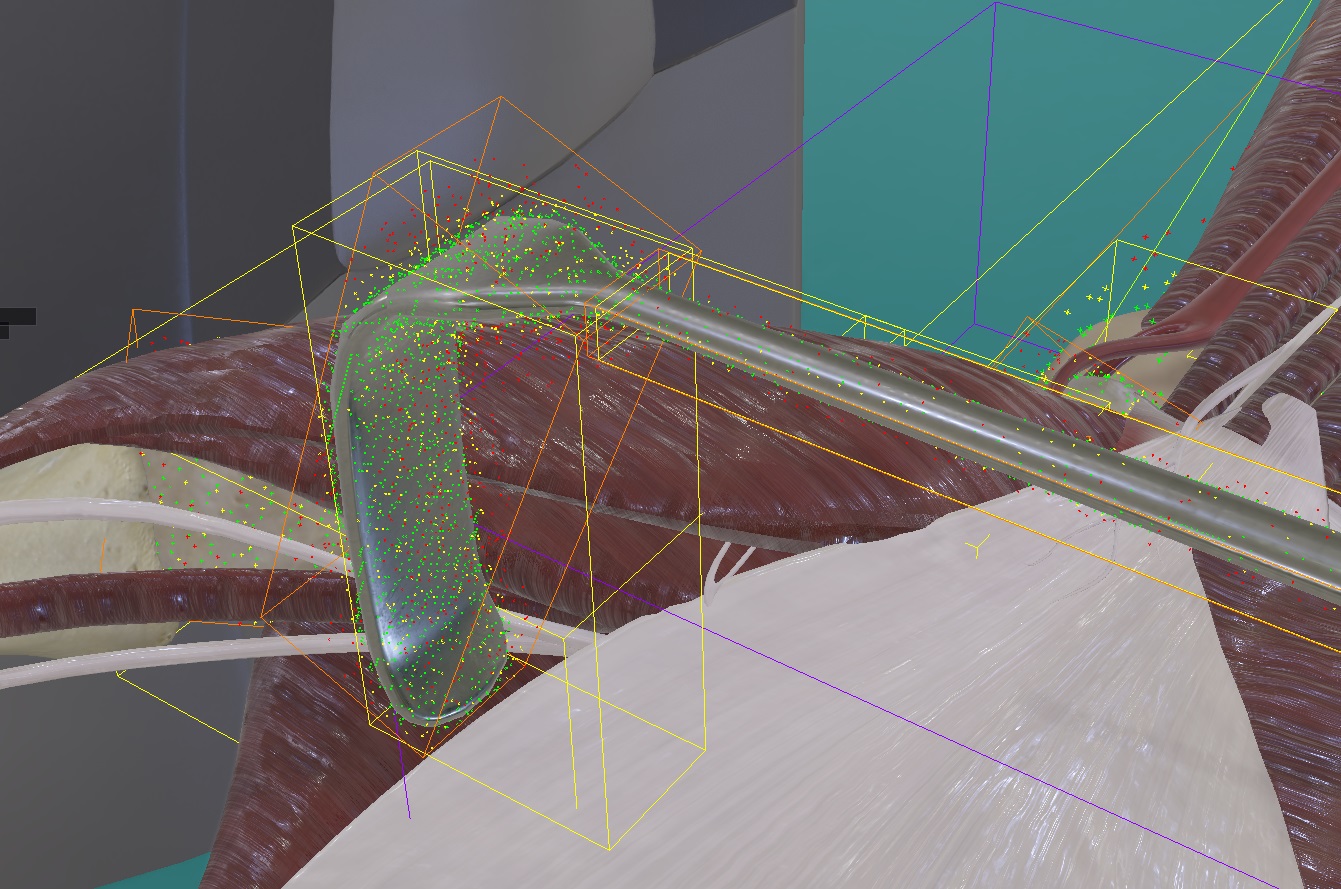
result<string_ view> stim:: simulation:: debug_dump_profiler() const noexcept
Dumps out the last snapshot of the simulation's profiler timing data.
Returns | A result<> containing the string dump, or an error message detailing why the string dump failed. |
---|
result stim:: simulation:: debug_ui(debug_ ui_ interface& ui,
debug_ ui_ groups groups = debug_ui_groups:: defaults) noexcept
Displays a debug user interface.
Parameters | |
---|---|
ui | The debug_ |
groups | The UI groups to feature in the interface. |
Returns | A success result<> , or an error message detailing why the UI invocation failed. |
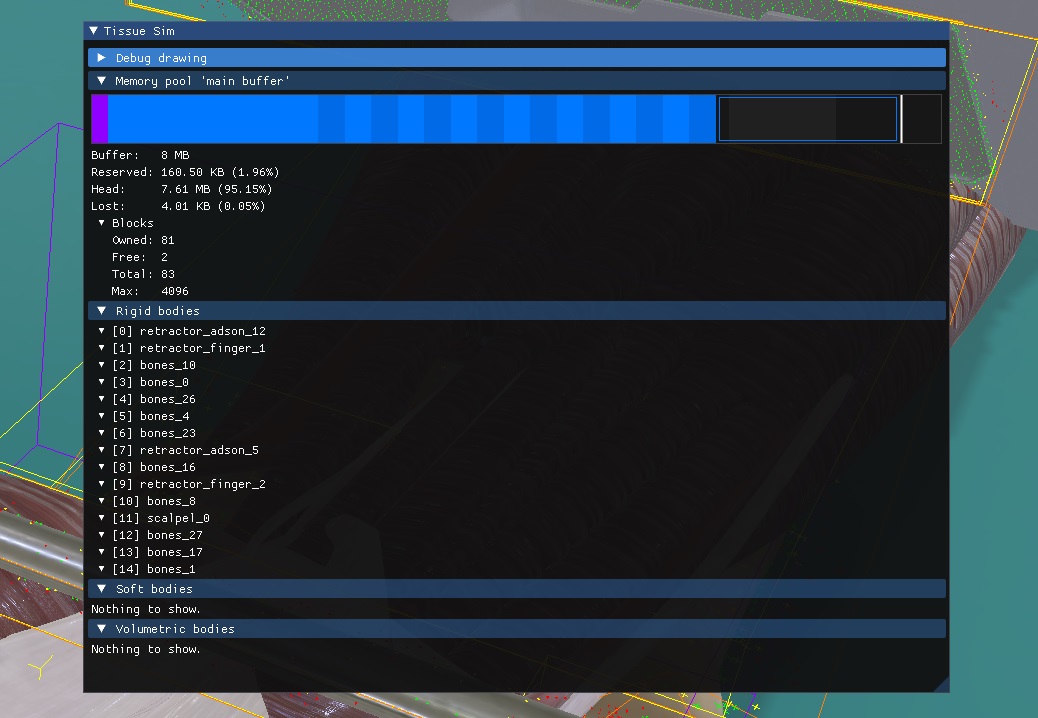
result<simulation> create_simulation(const simulation_ description& desc) noexcept
Creates a simulation.
Parameters | |
---|---|
desc | The simulation description. |
Returns | A new simulation, or an error message detailing why instantiation failed. |